用栈实现:输入一行符号,以#结束,判断其中的括号是否匹配。括号包括:
{} [] () <>
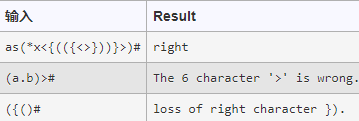
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144
| #include <iostream>
using namespace std; class steak; class StackNode { friend class LinkedStack;
public: StackNode *link; char data;
public: StackNode(StackNode *ptr = NULL) { link = ptr; } StackNode(char &c, StackNode *ptr = NULL) { data = c; link = ptr; } }; class LinkedStack { public: StackNode *top; public: LinkedStack(); ~LinkedStack() { makeEmpty(); } void Push(char &ch); int Pop(char &ch); char getTop(char &ch); int IsEmpty() { return (top == NULL) ? 1 : 0; } int getSize(); void makeEmpty(); void output(); };
LinkedStack::LinkedStack() { top = top = NULL; ; } void LinkedStack::makeEmpty() { if (top != NULL) { StackNode *p = top->link; while (top != NULL) { p = top; top = top->link; delete p; } } } void LinkedStack::Push(char &ch) { top = new StackNode(ch, top); } int LinkedStack::Pop(char &ch) { if (IsEmpty()) return 0; StackNode *p = top; top = top->link; ch = p->data; delete p; return 1; } int LinkedStack::getSize() { if (IsEmpty()) return 0; else { int a = 0; StackNode *p = top; while (p != NULL) { a++; p = p->link; } return a; } } int main() { LinkedStack s; char ch, ch1; cin >> ch; int num = 0; while (ch != '#') { num++; if (ch == '{' ch == '[' ch == '(' ch == '<') { s.Push(ch); } else if (ch == '}' ch == ']' ch == ')' ch == '>') { s.Pop(ch1); if (!((ch == '}' && ch1 == '{') (ch == ']' && ch1 == '[') (ch == ')' && ch1 == '(') (ch == '>' && ch1 == '<'))) { cout << "The " << num << " character '" << ch << "' is wrong."; return 0; } } cin >> ch; } if (!s.IsEmpty()) { cout << "loss of right character ";
while (!s.IsEmpty()) { s.Pop(ch1); if (ch1 == '{') { cout << "}"; } else if (ch1 == '[') { cout << "]"; } else if (ch1 == '(') { cout << ")"; } else if (ch1 == '<') { cout << ">"; } } cout << "."; } else { cout << "right"; } return 0; }
|